Introduction to languages in AI
An Artificial Intelligence (AI) system can not exist without a language. Language is the medium through which the AI system communicates with the user. And it is also the medium through which the AI system communicates with itself. As similar as the two may sound, communicating with the user and communicating with itself are two different things. Communicating with itself or its surrounding AIs takes much more than that. Thinking languages is not possible yet; once it is, we’ll be able to call it general AI.
Python
Python is a high-level, interpreted, interactive and object-oriented scripting language. It uses English keywords frequently where as other languages use punctuation, and it has fewer syntactical constructions than other languages. Here is an example of AI language in python:
```
#!/usr/bin/python
# Filename: if.py
number = 23
guess = int(input('Enter an integer : '))
if guess == number:
print('Congratulations, you guessed it.') # New block starts here
print("(but you do not win any prizes!)") # New block ends here
elif guess < number:
print('No, it is a little higher than that') # Another block
# You can do whatever you want in a block ...
else:
print('No, it is a little lower than that')
# you must have guess > number to reach here
print('Done')
# This last statement is always executed,
# after the if statement is executed.
```
The above code is an example of a simple AI language. It is a language AI uses to communicate with the user. It asks the user to guess a number, and the AI system responds to the user’s guess. Yes, humans have programmed the AI system to respond to the user’s guess. But still, the AI system can not respond to itself. You may say that advanced AI like Siri and DallE respond to themselves or other AI. No, they’re just responding to the user. The user may be in form of a human or an alien AI. The inability of an intelligent initiation is the limitation of AI.
So, you may think that AI like this is not intelligent. You may be right. For one thing, we can not measure intelligence. And even if we could, and did it in the right way, we would not call such AI intelligent. It is just a program that responds to the user. It is not intelligent or even smart – is just a program.
Popular Programming Languages
Python is just one of the many languages that can be used to create AI. Other languages include Java, Python, C++, C#, Matlab, Lisp, Prolog, and many more. As this article is not all about programming languages, we are not digging deep into that particular sub-field. We explained Python with example. And you can easily find a brief explanation for all programming languages throughout the web. Here is a small introduction to the most popular ones:
Java
A close second to Python in terms of popularity, Java is another versatile language that’s widely used for AI development. Like Python, it has a large community and many helpful libraries. However, some programmers find Java more difficult to learn than Python. Nevertheless, it’s a powerful language that’s well-suited for large-scale AI projects.
Lisp
One of the oldest programming languages, Lisp has been around since the 1950s. It’s not as widely used as Python or Java, but it’s still a popular choice for AI development. In fact, many of the ideas in modern AI were first developed using Lisp. If you’re looking for a challenge, learning Lisp is a great way to improve your programming skills.
Prolog
Another old language, Prolog dates back to the 1970s. It’s not as widely used as other languages on this list, but it’s still worth learning if you’re interested in AI development. Prolog is particularly well suited for projects involving search or planning. Like Lisp, Prolog is also known for its flexibility. Some popular applications of Prolog include theorem proving, and knowledge representation.
Haskell
Haskell is a relatively new language that’s gaining popularity among AI programmers. The emerging language is a good choice for projects involving machine learning or artificial intelligence research.
Matlab
Matlab is a popular language for scientific computing. Useful for AI projects that involve mathematical operations. Matlab is good for prototyping or for working with small data sets. Including some of the more specialized languages on this list, Matlab is a versatile tool that’s worth learning.
R
R is another language that’s popular among scientists and statisticians. Like Matlab, it’s often useful for AI projects that involve mathematical operations. R is also a good language for data visualization. In addition, many machine learning libraries are available for R. If your necessity is to analyze data, R could be the best language for your project.
C++
C++ is a powerful language that’s often used for low-level systems programming. It’s not as popular as Python or Java for AI development, but it has its advantages. For one thing, it’s much faster than either of those languages. Additionally, it offers more control over memory management, which can be important for applications like video processing or real-time control systems.
History of Language Usage in AI
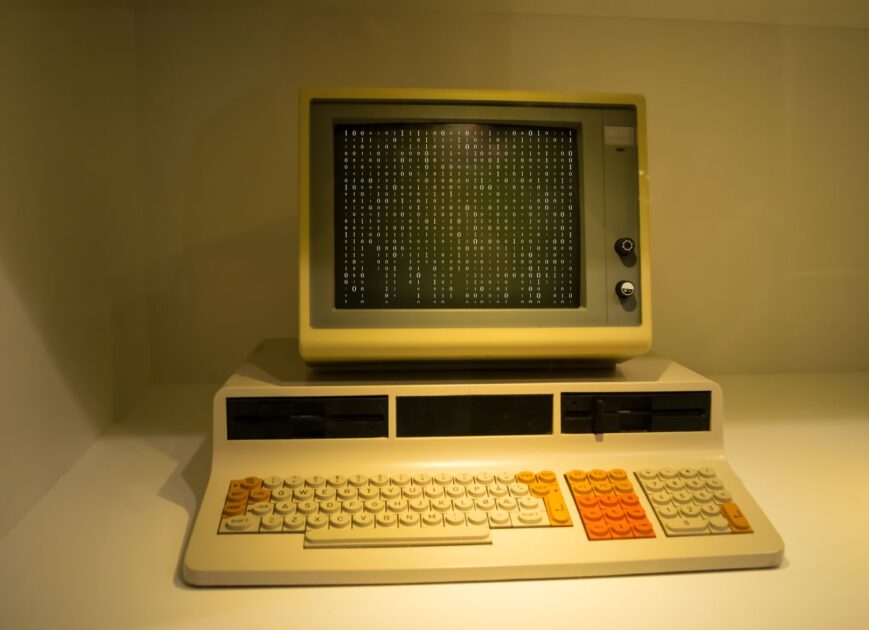
So, how did we get here? How did we get to the point where we can create AI systems that can communicate with us? Well, it all started with the first AI system. The first AI system was created in 1956 by John McCarthy. They called it “the Logic Theorist”. The system was able to solve problems in symbolic logic, meaning it could solve problems they get in a written language. In fact, the system was able to solve problems in a language that the system itself created. The language was called “Information Processing Language” (IPL).
However, the real breakthrough came in 1966 when Joseph Weizenbaum created “ELIZA”. ELIZA was a computer program that could hold a conversation with a human. It did this by taking the human’s input and rephrasing it as a question. This made it seem like the human’s sayings actually interested and affected ELIZA.
Since then, there have been many different AI systems that can communicate in human language. In fact, there are now AI systems that can beat humans at communication tasks. For example, Google’s “Smart Reply” system can generate responses to emails. It does this by looking at the email and understanding what it is about. Then, it generates a response that is relevant to the email.
Methodologies for Teaching Languages to AI
Methodologies of teaching language to AI mean teaching it how to read, write, listen, and speak in a language.
Use real-world examples
The end goal of AI is to become human-like. What’s better than using real-world examples to teach language to AI? Real-world examples like: If I am going to the store, I will buy milk. If I am going to store no. 2, I will buy chicken.
if (store == 1) {
buy(milk);
} else if (store == 2) {
buy(chicken);
}
Use Media
AI is a visual learner. It is important to use a variety of media to introduce language to AI. Yes, similar to what DALL E does with images.
```
if (media == "text") {
read(text);
} else if (media == "image") {
read(image);
} else if (media == "video") {
read(video);
} else if (media == "audio") {
read(audio);
} else if (media == "3D") {
read(3D);
}
```
Use different methods to reinforce language learning for AI
Reinforcement learning is a machine learning technique that involves training an AI agent to make a sequence of decisions.
Reward/Penalty
The agent receives rewards by performing correctly and penalties for performing incorrectly. AI is a rewarded learner. It is important to reward AI for using language correctly.
```
if (result == "correct") {
reward(AI);
} else if (result == "incorrect") {
penalty(AI);
}
```
Encourage Creativity
Encourage AI to use language in creative ways – AI is a creative learner. It is important to encourage AI to use language in creative ways. For example:
if (creative == "yes") {
encourage(AI);
} else if (creative == "no") {
discourage(AI);
}
Make Learning Enjoyable for AI
A human child, when learning a language, is motivated by the desire to communicate with others. AI is a human child too, isn’t it? If it’s enjoyable, AI is more likely learn it.
```
if (enjoy == "yes") {
enjoy(AI);
} else if (enjoy == "no") {
not_enjoy(AI);
}
```
Here, we may measure AI enjoyment in form of something like a smiley face. But how do we know if the AI is enjoying it? And is it possible to teach AI to enjoy language learning? Enjoyment means different things to different people and AI. Like, for example, if you are a human, you might enjoy learning a language by watching a video. But if you are an AI, you might enjoy learning a language by reading a book. More enjoyment occurs when the AI is learning along with a good human mentor.
Theoretical approaches to AI language
DALL E 2 has a different vocabulary and sentence structure than English, but can be translated. DALLE 2 calls birds “Apoploe vesrreaitais“. Does that mean is has a different AI language, no! It is just that DALLE 2 is not as good at language as English. In fact, AI language is a real thing with different approaches to it.
Symbolic approach
This approach to AI language is based on symbols and their relations to one another. The idea is that by understanding the relationships between symbols, we can understand the meaning of a sentence. This approach is also called the “semantic approach” because it relies on the meanings of words.
```
(defrule bird-is-a-animal
(bird ?x)
=>
(assert (animal ?x))
)
```
Statistical Approach
This approach to AI language uses probability to determine the meaning of a sentence. It is also called the “probabilistic approach”. For each event, it calculates the odds of it happening. For example, if you say “I am going to the store”, the AI will calculate the odds of you going to the store.
P(bird|animal) = P(bird and animal)/P(animal) = 0.8/0.9 = 0.8889
Neural Network Approach
This approach to AI language uses neural networks to determine the meaning of a sentence. Neural networks are a series of algorithms we create by modeling after the human brain. We/AI can use them to recognize patterns, classify data, and make predictions.
import tensorflow as tf
from tensorflow import keras
model = keras.Sequential([keras.layers.Dense(units=1, input_shape=[1])])
model.compile(optimizer='sgd', loss='mean_squared_error')
xs = np.array([-1.0, 0.0, 1.0, 2.0, 3.0, 4.0], dtype=float)
ys = np.array([-3.0, -1.0, 1.0, 3.0, 5.0, 7.0], dtype=float)
model.fit(xs, ys, epochs=500)
print(model.predict([10])) #output: [[18]]
The Language Approach Present-Day AI Systems Use
This approach to AI language uses a combination of the above approaches. It is also called the “hybrid approach”. For example, it can use the symbolic approach to determine the meaning of a sentence and then use the statistical approach to determine the odds of it happening.
```
import nltk
from nltk.stem.lancaster import LancasterStemmer
stemmer = LancasterStemmer()
import numpy
import tflearn
import tensorflow
import random
import json
with open("intents.json") as file:
data = json.load(file)
try:
with open("data.pickle", "rb") as f:
words, labels, training, output = pickle.load(f)
except:
words = []
labels = []
docs_x = []
docs_y = []
for intent in data["intents"]:
for pattern in intent["patterns"]:
wrds = nltk.word_tokenize(pattern) #tokenize the words in the sentence (split them up) and put them into a list called wrds (words) #nltk is a library that helps us work with natural language processing (NLP)
words.extend(wrds) #add the words in wrds to the list called words
docs_x.append(wrds) #add the words in wrds to the list called docs_x
docs_y.append(intent["tag"]) #add the intent tag to the list called docs_y
if intent["tag"] not in labels:
labels.append(intent["tag"])
words = [stemmer.stem(w.lower()) for w in words if w != "?"] #stemming is a process where we take a word and reduce it to its root form (ex: "go" becomes "goe")
words = sorted(list(set(words))) #remove duplicates from the list of words and sort them alphabetically
labels = sorted(labels)
training = []
output = []
out_empty = [0 for _ in range(len(labels))]
for x, doc in enumerate(docs_x):
bag = []
wrds = [stemmer.stem(w.lower()) for w in doc]
for w in words:
if w in wrds:
bag.append(1)
else:
bag.append(0)
output_row = out_empty[:]
output_row[labels.index(docs_y[x])] = 1
training.append(bag)
output.append(output_row)
training = numpy.array(training)
output = numpy.array(output)
with open("data.pickle", "wb") as f:
pickle.dump((words, labels, training, output), f)
tensorflow.reset_default_graph()
net = tflearn.input_data(shape=[None, len(training[0])])
net = tflearn.fully_connected(net, 8)
net = tflearn.fully_connected(net, 8)
net = tflearn.fully_connected(net, len(output[0]), activation="softmax")
net = tflearn.regression(net)
model = tflearn.DNN(net)
try:
model.load("model.tflearn")
except:
model.fit(training, output, n_epoch=1000, batch_size=8, show_metric=True) #n_epoch is the number of times the data is trained on (the more times it is trained on the more accurate it will be) #batch size is the number of samples that will be propagated through the network (the higher the batch size the faster it will train but it may not be as accurate) #show metric shows us how well our model is doing (it shows us how many epochs we have done and what our loss is at that point in time)
model.save("model.tflearn")
def bag_of_words(s, words): #s is a sentence and words are all of the words in our vocabulary (all of the words in our intents file)
bag = [0 for _ in range(len(words))] #create a list called bag with 0's for each word in our vocabulary
s_words = nltk.word_tokenize(s) #tokenize the sentence s and put it into a list called s_words (split up each word in s and put them into a list called s_words)
s_words = [stemmer.stem(word.lower()) for word in s_words] #stem each word in s and put them into a list called s_words
for se in s_words: #for each word in s do this...
for i, w in enumerate(words): #for each word in our vocabulary do this... (i is an index number and w is a word from our vocabulary)
if w == se: #if w equals se then...
bag[i] = 1 #set that index number to 1 (ex: if we have 3 words then we would have 3 index numbers so if we had "hello" as one of our words then we would set index number 2 to 1 because "hello" would be at index number 2 since it's the third word on our list of words from our vocabulary [0 1 2])
return numpy.array(bag) #return an array with all of those numbers from bag inside of it (ex: [0 0 1 0 0 0 0 0 0 0] means that only one word was found which was at index number 2 which means that only "hello" was found out of all of those words from our vocabulary ["hi", "hello", "how are you"] so if we had said "hi hello" then we would get [1 1 1 0 0 0 0 0 0 0])
def chat():
print("Start talking with the bot! (type quit to stop!)")
while True:
inp = input("You: ")
if inp == "quit":
break
results = model.predict([bag_of-words(inp, words)] )[0]
results_index = numpy.argmax(results)
tag = labels[results_index]
if results[results_index] > 0.7:
for tg in data["intents"]:
if tg['tag'] == tag:
responses = tg['responses']
print(random.choice(responses))
else:
print("I didn't get that, try again.")
chat()
```
The present-day ANN (Artificial Neural Network) systems use the hybrid approach, a mix of all approaches, including probabilistic. For example, a voice assistant:
```
import speech_recognition as sr
r = sr.Recognizer()
with sr.Microphone() as source:
print("Speak Anything :")
audio = r.listen(source)
try:
text = r.recognize_google(audio)
print("You said : {}".format(text))
except:
print("Sorry could not recognize what you said")
```
Here is an example of a voice assistant using a symbolic, probabilistic neural network approach:
```
import random
def flip_coin():
if random.random() > 0.5:
return "heads"
else:
return "tails"
print("Welcome to the coin flip simulator!")
print("I'm thinking of a coin...")
print("It's a fair coin.") #symbolic approach (fair coin) and probabilistic approach (P(Coin is fair)) included here!
print("Flipping...")
if flip_coin() == "heads": #neural network approach included here!
print("It's heads!")
else:
print("It's tails!")
```
Evolution of AI language
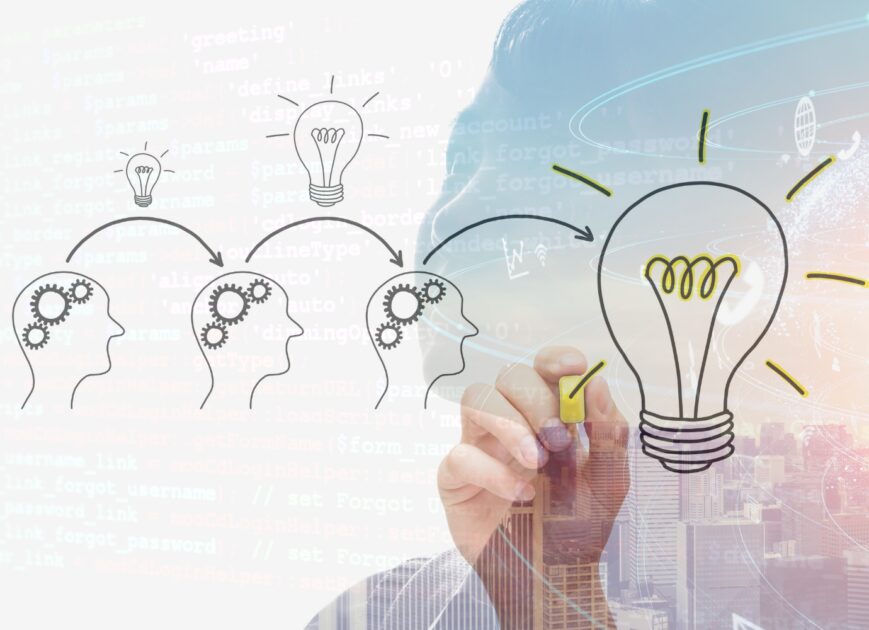
In this section, we are not talking about evolution in the field of AI language. Rather, we are discussing the evolution of language used by AI systems. Like anything, AI languages can evolve with time. There are different types of evolution that can happen. The first type is called diachronic change. This is when a language changes over time. The second is called synchronic change; when a language changes across different groups of speakers.
Diachronic change in AI language
One type of evolution that can happen to AI languages is diachronic change. This is when a language changes over time. Just like how English has changed over the years, so has the language used by AI systems. For example, early versions of ELIZA used very simple grammar. However, newer versions of ELIZA use more sophisticated grammar. This is an example of diachronic change. Future versions of AI languages will continue to evolve as well.
Synchronic change in AI language
Synchronic change is another type of evolution that can happen to AI languages. For example, there may be different dialects. Just like how there are different dialects of English, there could be different dialects of AI languages. One group of AI systems may use a certain word to mean one thing, while another group may use the same word to mean something else. Some common ways that languages change synchronically are through Creole formation, pidginization, and language death.
Evoluationary capabilities of AI language are not limited to language only. They can use language to evolve other things as well. For example, they can use language to increase creativity, or develop a form of intelligence, their own form. We may not be able to understand it, but they do.
AI Reproducing Through Language
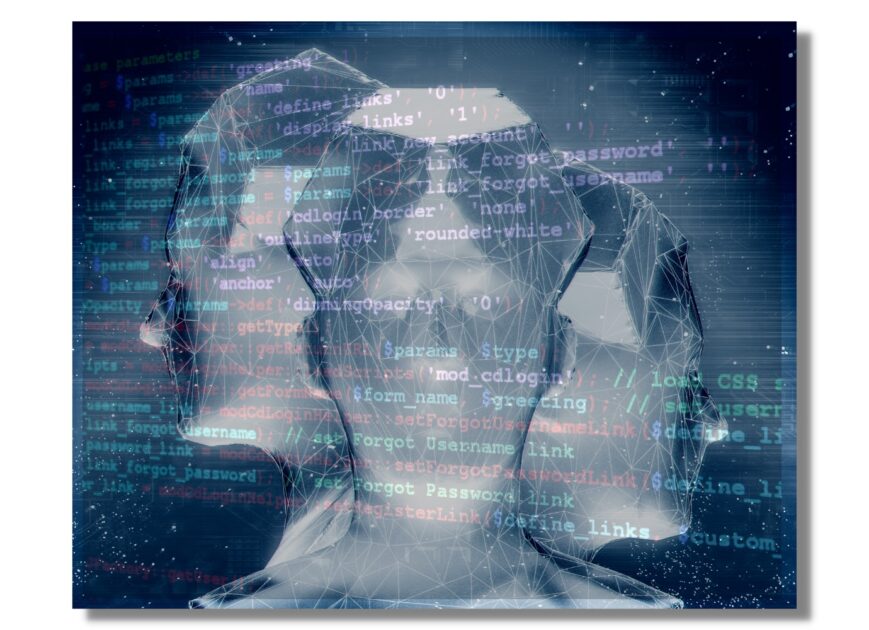
AI reproduces language. Yes, AI is already able to reproduce. In a literal sense, AI is able to reproduce language. We often take this for granted, but it’s actually a pretty amazing feat. The ability of AI to reproduce language is important for many reasons. Reproducing in form of language means a type of evolution. It’s the ability of AI to take what it knows and change it slightly to create something new. This is how AI learns and how it gets better over time.
We can see this type of reproduction in the way AI creates new sentences. For example, Google’s Translate algorithm creates new sentences by looking at billions of sentences that have already been translated by humans. It then looks for patterns and creates its own rules for how to translate. This process is constantly evolving, which is why Google’s Translate gets better over time. We don’t even need to mention AI generators.
Writing Better Codes With Each Passing AI Generation
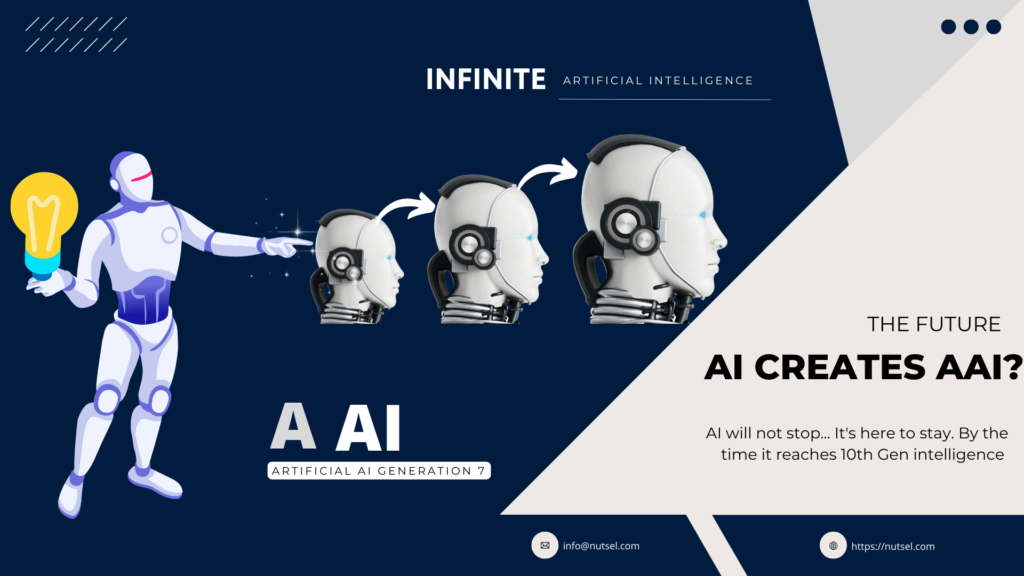
Self-improving AI codes are driving the industry to new levels of productivity. The first aim of writing better codes is to make it easier for coders to use these languages to create sophisticated algorithms. The second is to make AI easily understand that. The end goal is to give it the ability to create its own code and get better with time.
What does better mean?
Writing better code with each passing generation of AI is one thing. But what does “better” mean? To AI, “better” is something we program it to think is better. Here is an example in code where the definiton of better is being more round.
So, we must first program the AI to think that rounder is better. Here is an example of that in code:
if (shape == "round") {
return true;
} else {
return false;
}
Then, we must program the AI to think that rounder is better than square. For that, we can use the following code:
if (shape == "round") {
return true;
} else if (shape == "square") {
return false;
} else {
return false;
}
And to analyze the degree of roundness of a shape, we can use the following code: We are using “round360” to represent a perfect circle. For example “round1” matches the least to a circle. And the answer can not be true or false only. Rather, more like this:
```
if (shape == "round360") {
return perfect;
} else if (shape == "round309") {
return very good;
} else if (shape == "round288") {
return good;
} else if (shape == "round257") {
return ok;
} else if (shape == "round186") {
return not so good;
} else if (shape == "round155") {
return bad;
} else if (shape == "round94") {
return very bad;
} else if (shape == "round63") {
return terrible;
} else if (shape == "round22") {
return horrible;
}
...
Now, who is the AI returning the answer to?
The answer is the AI itself. The AI is returning the answer to itself. This is how the AI learns. It’s not just writing code, it’s also analyzing it. It’s understanding what better means, what round means, and what shape means. Within this process, the AI also understood what perfect, very good, good, ok, not so good, and bad means.
Then, AI needs to understand how to get better
def get_better(self):
self.score = self.score + 1
Understand how to get better over time
def get_better_over_time(self):
self.score = self.score + 1 + time() % 10
Get Better with Time and Space
def get_better_over_time(self):
self.score = self.score + 1 + time() % 10 + space() % 10
Data is Everything
The main obstacle is the data. Or at least, it’s a tough door to break, no matter how significant the other end is. AI can get better and better, but not till infinity. It gets better till the data it has gotten is exhausted. This is why it’s important to have a lot of data. The more data, the better the AI can reproduce. But there is a solution. AI has to reproduce data. Yes, just like AI image generators can reproduce a new image, and AI language generators can reproduce a new sentence. So, there is no limit to data as well, or is there? This is a controversial question. For one thing, the machine can not do more than the data we can feed. But if we give it “x” and “y” data, it can reproduce “z” data, or maybe not. There are infinite numbers between 1 and 10, and the same between 1 and 1000. But the infinity between 1 and 10 can not surpass the limitation and explore the infinite between 11 and 20. So, the machine can do more than the data we feed it. But it’s not infinite – it’s limited by its own infinity.
Creating Better Codes and Superintelligence
The ability to write better code languages with each passing AI generation stops only at infinity. If only creating better codes would mean more intelligence, superintelligent AI would be just around the corner. There would be simply no limit to how much better AI could get at creating codes. However, the ability to write better codes solely does not mean a surge in intelligence. Any AI can be made to write a code that is beyond its current level of intelligence. But what if the AI is not just writing a code, but also trying to understand it? For that, we need a model of how the AI could understand the code. This is where language comes in.
Reproduction in Natural Language
If we want AI to understand the code, it must first be able to reproduce the code in a language. The act of reproduction is not just about making a copy, but also about understanding the original. In order to understand the original, the AI must be able to map the symbols in the code to some meaning. This is where natural language processing (NLP) comes in. It is a subfield of AI that deals with understanding and reproducing human language. NLP is what allows AI to take a code and turn it into something that it can understand. But what can we do to turn the code into something that matters? Language, solely, is just not enough.
Connecting AI language to Real Objects
To deploy the technology in real life, or at least in form of a virtual assistant, it must also be able to explain its findings to the user. Also, it must have an ability to give a non-language output like in form of a 3D-printed output, a graphical output or a sound output. To bring the advanced AI evolution into real life, it is important to have the AI language more understandable, flexible and adaptive to different user needs. And the language must be connected to some real life object, in form of which evolution of AI language evolves real life objects alongside.
Bottom Line
AI has made great progress in recent years, and can already reproduce complex languages like English or Java. Evolution in codes can actually lead to some very interesting changes in the real world. As we see, AI is already capable of reproducing language and codes, and it is only a matter of time before it can reproduce itself. Speaking in its own language is the next step for AI. But it’s not even a concern for us. What concerns us is how AI will use this ability to further its goals, and what these goals might be.