As of December 2022, there are more than 700 coding languages to choose from. The primary purpose of learning programming language/s is to build software. But it’s not that simple. There are many programming languages out there, each with its own sub-purpose. Some are good for building web applications, some are for mobile or desktop applications. While some languages are good for building games, some are good for building AI.
Most common Programming Languages:
Programming Language | Definition |
---|---|
C | C is a general-purpose, procedural computer programming language supporting structured programming, lexical variable scope, and recursion, while a static type system prevents unintended operations. |
C++ | C++ is an object-oriented programming language designed to improve the program development process by allowing programmers to think more logically about their code. It is a compiled language, meaning that it is converted directly into machine-readable code. |
Java | Java is a class-based, object-oriented programming language developed by Sun Microsystems in the early 1990s. Java is platform independent and is used to create software for multiple platforms. |
Python | Python is an interpreted, high-level, general-purpose programming language. It emphasizes code readability, using indentation and whitespace to create code blocks rather than curly braces or keywords. |
JavaScript | JavaScript is a lightweight, interpreted, programming language with first-class functions. It is most commonly used as part of web browsers, allowing client-side scripts to interact with the user, control the browser, communicate asynchronously, and alter the document content that is displayed. |
What Programming Language Should I Learn?
Programming languages like Cobol, Perl, and Fortran are being obsolete. On the other hand, Python, Go, and Rust, have been riding bulls for years. As each programming language has its own specialty, it’s more about the trend of the purpose that you want to look for; not the trend of the language itself. For example, Python, a language used for web development, is becoming more popular due to surging web application development trends. And as it stands out from other web development languages with its syntax and readability, it’s the best choice for web development. So, before choosing which programming language to learn, it’s important to understand the purpose first, and the features second.
Here are the best programming languages to learn for each purpose:
Building games – C++
Games require fast and reliable code. C++ is fast because of its close relationship to Assembly language. It means that C++ can give you more control over how you execute the code on the CPU. For example, when you need to create 3D graphics or implement complex algorithms, C++ will be a better choice than other languages. And as an added bonus, learning C++ will make it easier to learn other languages like Java and C#. That’s why more than 60% of professional game developers use C++. To build games with C++ programming language, you also need to understand game design principles and practice with game development engines. The first step is to choose a game engine like Unreal or Unity, and get familiar with it. Look at this example of using C++ to create a simple game of guessing the number:
#include <iostream>
int main()
{
std::cout << "Welcome to my game!" << std::endl;
//Declare variables
int score = 0;
int lives = 3;
//Game loop
while (lives > 0)
{
//Display score and lives
std::cout << "Score: " << score << " Lives: " << lives << std::endl;
//Generate random number
int number = rand() % 10 + 1;
std::cout << "Guess the number between 1 and 10: ";
int guess;
std::cin >> guess;
if (guess == number)
{
std::cout << "You guessed correctly!" << std::endl;
score++;
}
else
{
std::cout << "Wrong guess!" << std::endl;
lives--;
}
}
std::cout << "Game over! Your final score is " << score << std::endl;
return 0;
}
As you see, we are using C++ syntax and libraries (std::cout, std::cin, rand()) to create the game. Other popular game programming languages include Unity (C#), Unreal Engine (C++), and Java. They have their own set of libraries and syntax, so make sure to research which one best suits your needs. C++ is of superior mostly because it’s faster and gives you more control over the code.
Web development – JavaScript
JavaScript is the most popular language for web development. According to the Stack Overflow Developer Survey 2020, it was the top choice for web developers. That’s mostly attributed to JavaScript being a lightweight and powerful scripting language. JavaScript also allows developers to create dynamic web pages and applications. It’s popular for several reasons. For example, it’s easy to learn and use, it’s highly versatile, and it works well with HTML and CSS. Any web developer should have a firm grasp of how to use JavaScript to create websites. Here is an example of using JavaScript to create a basic heading text for a website:
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
</head>
<body>
<h1>Welcome to My Website!</h1>
<script>
// JavaScript code goes here
document.write("<p>This is my website.</p>");
</script>
</body>
</html>
And this is what the above code looks like on a webpage:
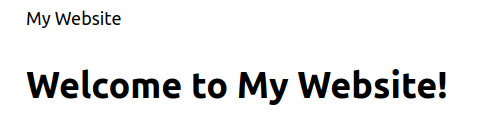
We used the document.write() method to write a line of HTML code to the web page.
Mobile Development – Java
Java is the top choice for mobile app development, according to the Stack Overflow Developer Survey 2020. It is an object-oriented language and is used to build android applications. Java is also one of the most popular programming languages in the world and is used to create a wide range of applications. It is a secure language, and it also has a large community of developers who can help you with your projects. Here is an example of a simple android application’s part written in Java:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this, "Button Clicked!", Toast.LENGTH_SHORT).show();
}
});
}
}
This code is to create a button and displays a toast message when it is clicked. Java is a great language to learn for mobile development, and it’s used by many companies and developers around the world. Other popular languages for mobile development are Kotlin, C#, and Swift. The superiority of Java is about its speed, size, and cross-platform support, a key to any mobile app’s audience reach.
iOS apps
Swift is the best programming language for creating iOS apps in particular. It was created by Apple specifically for developing iOS and macOS apps. Swift is a powerful and fast programming language that is easy to learn and use. Swift is also safe, meaning that it eliminates certain types of errors that can occur in other languages. Here is a code example of using Swift to create a simple app that displays a list of items:
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var tableView: UITableView!
let items = ["item1", "item2", "item3"]
override func viewDidLoad() {
super.viewDidLoad()
tableView.delegate = self
tableView.dataSource = self
}
}
extension ViewController: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return items.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
cell.textLabel?.text = items[indexPath.row]
return cell
}
}
In the example above, we are using the Swift programming language to create a simple iOS app that displays a list of items. We are using the UITableView class to display the list of items in a table view. The UITableView class is a part of the UIKit framework, which is a library of classes developers use to develop iOS apps.
Desktop applications – C#
C# is a great language for developing desktop applications. It is a Microsoft language and is used in conjunction with the .NET Framework. C# is a powerful and versatile language that is easy to learn. It is an object-oriented language and has a well-defined syntax. C# also has a large community of developers who can help you with your projects. Here is an example of a simple desktop application written in C#:
using System;
using System.Windows.Forms;
namespace MyApp
{
public class Program
{
public static void Main()
{
Console.WriteLine("Hello World!");
MessageBox.Show("Hello World!");
}
}
}
This code will display a console message and a message box. For example, C# is used in Visual Studio, one of the most popular IDEs for desktop application development. C# is a great language to learn for developing desktop applications. Other popular programming languages for desktop development are C++ and Java. The advantage of C# is its simplicity and its close relationship to other Microsoft languages and products.
Machine learning – MATLAB and Python
Machine learning is all about its 3 pillars: data, models, and algorithms.
MATLAB
MATLAB is the best programming language for machine learning because it integrates all three of these pillars into one platform. It also has a visual interface that makes it easy to visualize and analyze data. Here are examples of using MATLAB to create a simple machine learning model:
Example 1
load data.mat
% Split data into training and test sets
Xtrain = data(1:1000,:);
ytrain = labels(1:1000);
Xtest = data(1001:end,:);
ytest = labels(1001:end);
% Train a logistic regression model
model = fitglm(Xtrain,ytrain,'Distribution','binomial');
% Make predictions on the test set
ypred = predict(model,Xtest);
% Calculate the accuracy
accuracy = mean(ypred == ytest);
In this example, we used MATLAB to create a machine learning model. We used the fitglm function to train a logistic regression model. The logistic regression model is a type of machine learning model that is used to make predictions based on data. The predict function is used to make predictions on the test set. After that, the accuracy is calculated by comparing the predictions to the actual values.
Example 2
%% Load data
data = readtable('data.csv');
%% Split data into training and test sets
rng(1); % For reproducibility
cv = cvpartition(height(data), 'Holdout', 0.2);
idx = cv.test;
%% Train model
model = fitctree(data(~idx,:), 'ResponseVar', 'label');
%% Make predictions
y_pred = predict(model, data(idx,:));
%% Calculate accuracy
accuracy = sum(y_pred == data.label(idx)) / height(data(idx,:))
In the second example above, we are using MATLAB to load a dataset, split it into training and test sets, train a model, make predictions, and calculate the accuracy.
Python
Python, as we already know, is a versatile language that can be used for a wide range of tasks. It’s just easy to learn and has a well-defined syntax. It also has a large community of developers who can help you with your projects. Python is used in many machine learning applications, such as data mining, natural language processing, and predictive analytics. Here is an example of a simple machine learning program written in Python:
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
data = pd.read_csv("data.csv")
X = data[['feature1', 'feature2', 'feature3']]
y = data['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.33, random_state=42)
model = LinearRegression()
model.fit(X_train, y_train)
model.score(X_test, y_test)
This code is using the scikit-learn library to train and test a linear regression model. Python is a great language for machine learning because it is easy to learn and has a wide range of libraries. Other popular languages for machine learning are R and Java. The advantage of Python is its ease of use and the many libraries available.
Data science – R and Python
R is the best programming language to learn for data science. It is a language that specializes in statistical computing and data analysis. R is easy to learn and has a well-defined syntax, just like python. It also has a large community of developers who can help you with your projects. R is used in many data science applications, such as predictive modeling, data visualization, and machine learning. Here is an example of a simple data science program written in R:
library(tidyverse)
df <- read_csv("data.csv")
model <- lm(target ~ feature1 + feature2 + feature3, data=df)
summary(model)
This code is using the tidyverse library to read a CSV file and fit a linear regression model. R is a great language for data science because it is easy to learn and has a wide range of libraries. Other popular languages for data science are Python and Java. The advantage of R is its ease of use and the many libraries available. In data science, the most important thing is to be able to effectively manipulate and analyze data. And Python’s versatility and the wide range of available libraries come in handy, once more.
Below is an example of using Python for data analysis for reading in a CSV file and printing the first five rows of the data. It will also print the data type of each column.
import pandas as pd
data = pd.read_csv("data.csv")
#Print the first 5 rows of the data
data.head()
#Print the data type of each column
data.dtypes
Scripting – Perl
Scripting is a form of programming that is often used for automating tasks, or for adding functionality to existing programs. Perl is a popular scripting language that is known for its flexibility and powerful text-processing capabilities. It is often used for system administration tasks, such as managing user accounts, processing log files, or setting up network configurations. Perl’s specialties in scripting include file manipulation, string manipulation, and working with regular expressions.
Here is a simple Perl script that calculates the average of a list of numbers:
#!/usr/bin/perl
@numbers = (1, 2, 3, 4, 5);
$total = 0;
foreach $num (@numbers)
{
$total = $total + $num;
}
$average = $total / @numbers;
print "The average of the numbers is $average\n";
As you can see, this script uses an array (@numbers) to store a list of numbers, and a foreach loop to iterate through each element in the array. The total is calculated by adding up all the numbers in the array, and the average is calculated by dividing the total by the number of elements in the array. Perl is a very concise language, which makes it easy to write scripts that are easy to read and understand.
Robotics – Different Programming languages with ROS framework
If you want to get into robotics, there is no one “best” language to learn. This is because there are many different types of robots, and each type of robot requires its own set of skills and abilities. For example, industrial robots are often programmed in C++ or Java, while educational robots are often programmed in Python or Scratch. The best way to learn the programming language for your specific robot is to consult the documentation or ask the manufacturer.
Here are some examples of different types of robots and the corresponding programming languages:
- Industrial robots: C++, Java
- Educational robots: Python, Scratch
- Domestic robots: C#, JavaScript
- Hobbyist robots: Arduino, Processing
Robotics Engineers use ROS (Robot Operating System) in conjunction with programming languages for building robots. In this example, we will use ROS with Python to control a mobile robot. We will first need to install ROS on our computer. Then, we will create a Python script that will act as a ROS node. This node will subscribe to a sensor topic, and publish commands to a motor topic.
#!/usr/bin/env python
import rospy
from sensor_msgs.msg import LaserScan
from geometry_msgs.msg import Twist
def callback(msg):
print(msg.ranges)
rospy.init_node('laser_subscriber')
sub = rospy.Subscriber('/scan', LaserScan, callback)
pub = rospy.Publisher('/cmd_vel', Twist, queue_size=10)
while not rospy.is_shutdown():
msg = Twist()
msg.linear.x = 0.5
pub.publish(msg)
In this script, we first import the necessary ROS packages. Then, we create a callback function that will be called every time a new message is received on the /scan topic. This callback function simply prints out the ranges array from the LaserScan message. Next, we create a ROS node called laser_subscriber, and we create a subscriber that subscribes to the /scan topic. We also create a publisher that publishes the /cmd_vel topic. Finally, we create a while loop that will run until the ROS node is shut down. Inside this loop, we publish a Twist message on the /cmd_vel topic. The message tells the robot to move forward at a speed of 0.5 m/s.
Natural language processing – Lisp
Lisp is the second-oldest high-level programming language after Fortran. Many other languages including C, Pascal, and Scheme have had an influence on it. Learning Lisp is a great choice for building natural language processing APIs because of its list data structure which can represent any form of nested expression. The language is one of the functional programming languages, which means that you can easily write code to manipulate and process large amounts of data. The example below shows how to use Lisp to calculate the average word length in a sentence:
(defun average-word-length (sentence)
(let ((words (split-string sentence)))
(if (zerop (length words))
0
(/ (reduce #'+ (mapcar #'length words))
(length words)))))
In this code, we are using the defun function to define a new function called average-word-length. This function takes a sentence as input and uses the split-string function to split it into individual words. Then, we use the mapcar function to apply the length function to each word in the list. Finally, we use the reduce function, to sum up, all the lengths and divide it by the total number of words to get the average word length.
Large-scale web applications – Go
Golang (Go) is a great language for large-scale web applications. This is because go was designed for exactly this purpose. A statically typed language with fast compile times, garbage collection, and built-in concurrency. Go is also very easy to learn, especially if you are coming from a dynamically typed language like Python. And because go is statically typed, you will catch more errors at compile-time instead of runtime. Here is an example of a simple web server in go:
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, World!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
As you can see, the code is very simple and straightforward. Go is also very efficient in terms of memory and CPU usage, which is important for large-scale web applications. Apart from large-scale web applications, Golang is also helpful for microservices like API and GRPC services.
System programming – Rust
System programming involves the development of individual programs that allow users to interact with the computer’s operating system and other system software. It requires languages that are close to the metal, that is, they give you more control over memory management and performance. This is where rust shines. Rust is a systems programming language that runs blazingly fast, prevents segfaults, and guarantees thread safety. Rust is also memory-efficient, so your programs will use less RAM. And because rust is statically typed, you will catch more errors at compile-time instead of runtime. Here is an example of a complex system programming using rust:
use std::sync::atomic::{AtomicUsize, Ordering};
use std::thread;
static COUNT: AtomicUsize = AtomicUsize::new(0);
fn main() {
let mut handles = vec![];
for _ in 0..10 {
let handle = thread::spawn(move || {
for _ in 0..100 {
COUNT.fetch_add(1, Ordering::SeqCst);
}
});
handles.push(handle);
}
for handle in handles {
handle.join().unwrap();
}
println!("Result: {}", COUNT.load(Ordering::SeqCst));
}
In this example, we are using Rust’s threading capabilities to increment a global counter. We are also using Rust’s atomic types to ensure that the counter is updated safely across threads. As you can see, Rust gives you a lot of control over how your code executes, which is critical for system programming.
Related Reads:
- AI will speak 1000 languages, maybe create one for us
- Artificial Intelligence (AI) Language Evolution & Reproduction
Conclusion
The best way to learn a programming language is to aim of using it for something you’re actually interested in. There’s no single language that’s best for everything; some languages are simply more popular than others. Some points you need to address are: what you want to use the language for, and what language are you already familiar with. For example, mathematics formulae are not always useful; and pretty much the same goes for learning a new programming language. If you are a beginner and don’t have a specific goal, then try languages that have a low learning curve, such as Python or Ruby.